What is JavaScript Rendering?
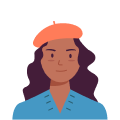
Specialist in Anti-Bot Strategies
JavaScript rendering is a fundamental process in modern web development, where JavaScript code dynamically updates or creates content on web pages. This technique is essential for creating interactive, user-friendly websites and is particularly prevalent in Single Page Applications (SPAs), which rely heavily on JavaScript to load new data without requiring a full page reload. JavaScript rendering not only enhances user experiences but also introduces complexities in web scraping, as it requires special techniques to capture dynamically loaded content.
How JavaScript Rendering Works
JavaScript rendering is the process where the browser executes JavaScript code to build and update the visible content of a web page. This process is common in Single Page Applications (SPAs) and dynamic websites that rely on JavaScript to fetch, update, and display data in real time. Here’s a breakdown of the main steps involved:
1. Initial HTML Request and Minimal Content Loading
When a user requests a page (e.g., by entering a URL or clicking a link), the browser makes a request to the web server. For JavaScript-heavy websites, the server often sends a basic HTML structure with minimal content, typically including placeholders for where data will be dynamically loaded. This initial HTML might only contain a framework skeleton with essential tags and references to external JavaScript files.
2. JavaScript Files and Resources Loading
After the initial HTML loads, the browser begins downloading JavaScript files and other resources like CSS (for styling) and images. These JavaScript files usually contain the code responsible for dynamically loading and rendering the remaining content.
3. Executing JavaScript and Fetching Dynamic Data
Once the JavaScript code is loaded, the browser executes it. In many cases, the JavaScript code will make asynchronous requests, such as AJAX (Asynchronous JavaScript and XML) calls, to retrieve additional data from APIs or other endpoints. This asynchronous approach allows the page to update without requiring a full page reload.
4. DOM Manipulation and Content Rendering
As data is fetched, JavaScript uses it to update the DOM (Document Object Model), which represents the structure of the web page. JavaScript frameworks like React, Vue, or Angular often manage this process. JavaScript may add new HTML elements, update text, or change styles in the DOM, allowing content to appear dynamically.
5. User Interaction and Further Updates
With JavaScript-rendered pages, interactions can trigger further content updates without reloading the page. For instance, clicking a button may prompt the JavaScript to fetch new data and update the page in real-time, providing a seamless and interactive experience.
What is the Difference Between HTML and JavaScript Rendering?
The primary difference between HTML and JavaScript rendering lies in how content is loaded and displayed:
-
HTML Rendering: This is the traditional rendering method where the server sends a fully constructed HTML document, and the browser displays it immediately. The content is static, meaning it doesn’t change without a full page reload. HTML rendering is simple and efficient, making it ideal for static content.
-
JavaScript Rendering: In contrast, JavaScript rendering relies on JavaScript to load additional data and update the page dynamically after the initial HTML is loaded. This allows content to be interactive and dynamic but requires the browser to execute JavaScript to display the full content. JavaScript rendering is essential for applications needing a high level of interactivity, such as social media or e-commerce platforms.
Challenges in Web Scraping with JavaScript Rendering
For scrapers, JavaScript rendering introduces a significant challenge. Standard HTTP requests to the server return only the initial HTML and often exclude JavaScript-generated content. This limitation means that scrapers must either simulate a browser environment or use tools that support JavaScript execution to retrieve dynamically generated data.
Common Approaches to Handle JavaScript in Scraping:
- Headless Browsers: Tools like Puppeteer and Playwright are essential for handling JavaScript rendering in web scraping. These headless browsers function like a virtual user interacting with a website. They load the entire web page in the background—just like a regular browser—but without displaying the graphical interface. Once the page is loaded, they execute the JavaScript, which can manipulate the Document Object Model (DOM) to display dynamic content that may not be visible in the initial HTML response. This capability allows scrapers to capture fully rendered pages, including content loaded via AJAX requests or other client-side operations.
In addition, Scrapeless provides a powerful Scraping Browser that seamlessly integrates with these processes, making it easier for developers to extract data from complex, JavaScript-driven sites.
-
API Endpoints: Some websites offer APIs that provide data directly in JSON or XML formats, bypassing the need for JavaScript rendering. When available, APIs are an efficient way to obtain structured data without executing JavaScript.
-
AJAX Requests: Many websites use AJAX (Asynchronous JavaScript and XML) to fetch data asynchronously without reloading the page. By inspecting AJAX requests, scrapers can directly access these endpoints and retrieve the required data without the overhead of a headless browser.
Here’s a revised version of the section on avoiding detection while scraping, presented in a more narrative style with added detail:
How to Avoid Getting Blocked While Scraping?
When scraping JavaScript-rendered content, stealth is key to reducing the risk of being detected and subsequently blocked by the website. Websites employ various measures to identify and thwart scraping attempts, so employing effective strategies is crucial for successful data extraction.
One effective approach is to use rotating proxies. If you make frequent requests from a single IP address, it can quickly raise red flags. By utilizing a pool of rotating proxies, you can distribute requests across multiple IPs, mimicking the behavior of different users and making it harder for the website to detect scraping activity.
Another critical strategy is to throttle your requests. Rapid-fire requests can signal automated activity, so it's vital to space out your requests at intervals that closely resemble human behavior. For example, introduce random delays between requests to mimic the natural variability of human browsing patterns. This simple adjustment can significantly reduce the likelihood of detection.
In addition, consider randomizing your user agents. Many websites monitor incoming requests for default user-agent strings associated with popular scraping tools. By randomly changing the user-agent string with each request, you create a façade of diversity, simulating requests from different browsers and devices, which adds another layer of unpredictability.
When employing browser automation tools like Puppeteer or Playwright (will have more detail below), it's essential to act cautiously. Rapid page loads, repetitive actions, or unnatural scrolling can trigger detection mechanisms designed to identify bot-like behavior. Therefore, it’s wise to include deliberate pauses between actions and to interact with the page in a manner that feels organic.
Having trouble with web scraping challenges and constant blocks on the project you're working on?
Please use Scrapeless to make data extraction easy and efficient, all in one powerful tool.
Try it free today!
JavaScript Rendering in Action: Puppeteer and Playwright
Using headless browsers like Puppeteer and Playwright provides the most robust approach to handling JavaScript-rendered content. These tools allow scrapers to load pages as a real user would, execute JavaScript, and capture dynamic content. For instance, Puppeteer can emulate mouse clicks, type text, and scroll, enabling the scraper to interact with the page. This technique is essential for scraping content from SPAs (Single Page Applications) or websites that rely heavily on client-side rendering.
Example of Scraping with Puppeteer
Here’s an example of using Puppeteer to scrape JavaScript-rendered content:
javascript
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com', { waitUntil: 'networkidle2' });
// Wait for content to load and render
const content = await page.evaluate(() => document.querySelector('body').innerText);
console.log(content);
await browser.close();
})();
In this example, Puppeteer waits for the network to be idle (indicating content loading is complete) before extracting the text from the body of the page, capturing the fully rendered content.
Example of Scraping with Playwright
Similarly, Playwright is another powerful headless browser automation tool that enables efficient web scraping of JavaScript-rendered content. Below is an example demonstrating how to use Playwright for web scraping:
javascript
const { chromium } = require('playwright');
(async () => {
// Launch a headless Chromium browser instance
const browser = await chromium.launch();
// Open a new browser context and a page
const context = await browser.newContext();
const page = await context.newPage();
// Navigate to the desired URL and wait until the network is idle
await page.goto('https://example.com', { waitUntil: 'networkidle' });
// Extract the visible text from the body of the page
const content = await page.textContent('body');
// Log the extracted content to the console
console.log(content);
// Close the browser context and instance
await context.close();
await browser.close();
})();
Explanation of the Code
-
Launching the Browser: Both examples start by launching a headless browser instance (Puppeteer for Chrome and Playwright for Chromium).
-
Creating a New Context/Page: In Playwright, a new context is created to isolate sessions, while Puppeteer simply opens a new page in the default context.
-
Navigating to the URL: The scripts navigate to the specified URL with
waitUntil: 'networkidle'
, ensuring that all JavaScript content has been loaded before extraction. -
Extracting Content: Puppeteer uses
page.evaluate()
to execute JavaScript in the page context to retrieve the body text, while Playwright employspage.textContent()
to directly extract the inner text of the body element. -
Logging and Closing: Both scripts log the extracted content to the console and properly close their respective browser instances to free up resources.
Practical Applications
Using Puppeteer and Playwright for web scraping is particularly beneficial for extracting data from websites that depend heavily on client-side JavaScript. Their capabilities to automate interactions and handle multiple browsers make them versatile choices for developers looking to scrape data efficiently.
Key Advantages of JavaScript Rendering
JavaScript rendering brings significant benefits for web users, enhancing the speed and interactivity of websites. By delivering content dynamically, JavaScript allows web pages to update in real-time, creating smooth user experiences without constant page reloads. This responsiveness is especially valuable for websites that handle large data volumes or rely on personalized content, such as social media platforms, e-commerce sites, and news applications.
The Role of JavaScript Rendering in SEO
JavaScript rendering has implications for search engine optimization (SEO). Since search engine bots traditionally struggle with JavaScript execution, websites relying on client-side rendering may encounter issues in getting indexed accurately. Google has adapted by using a two-wave indexing process that includes rendering JavaScript content, but this process can introduce delays. To improve SEO, many sites opt for server-side rendering or hybrid models (SSR combined with CSR) to ensure essential content is available in the initial HTML response.
Conclusion
JavaScript rendering is a transformative feature in modern web development, enabling the creation of fast, dynamic, and interactive web applications. For developers, it brings flexibility and a responsive user experience, while for scrapers, it presents challenges that require advanced techniques like headless browsing and AJAX inspection. Understanding JavaScript rendering is essential for both creating and interacting with today’s web applications, particularly as the web continues to evolve towards increasingly dynamic and personalized experiences.
At Scrapeless, we only access publicly available data while strictly complying with applicable laws, regulations, and website privacy policies. The content in this blog is for demonstration purposes only and does not involve any illegal or infringing activities. We make no guarantees and disclaim all liability for the use of information from this blog or third-party links. Before engaging in any scraping activities, consult your legal advisor and review the target website's terms of service or obtain the necessary permissions.