What is DOM Manipulation? A Comprehensive Guide for Beginners
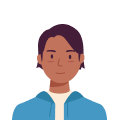
Expert Network Defense Engineer
DOM Manipulation is a fundamental concept for any web developer or web scraper looking to interact with or modify web pages dynamically. But beyond its basic definitions, DOM manipulation is a key component in modern web development, especially when working with frameworks like React. For scrapers and developers alike, a strong understanding of the DOM can streamline tasks, enable precise element targeting, and allow for more sophisticated interactions with web content.
This guide will unpack the core of DOM manipulation, its applications, particularly in React, and its differences from BOM (Browser Object Model), offering a well-rounded look at how these technologies work together to create dynamic, responsive, and interactive web experiences.
What is DOM Manipulation?
DOM (Document Object Model) Manipulation refers to the process of modifying or interacting with the structure, content, or styling of a web page through programming languages like JavaScript. The DOM is essentially a tree-like structure representing the HTML document, with each node in the tree representing a document element (such as headers, images, paragraphs, etc.). This structured representation allows developers to access, add, modify, or delete elements and attributes on a webpage dynamically.
For example, when you click a button on a webpage that opens a new section, or submit a form that updates the page without refreshing, these interactive experiences are often powered by DOM manipulation. JavaScript, with its extensive DOM API, enables developers to programmatically change the page's content and layout based on user interactions or other triggers.
Why is DOM Manipulation Important?
DOM manipulation is central to creating interactive and responsive web applications. Without it, web pages would be static and unresponsive to user actions. Some common scenarios where DOM manipulation plays a crucial role include:
- Updating content dynamically: Adding or changing text, images, and other elements without reloading the entire page.
- Handling user interactions: Listening to user actions like clicks, mouse movement, and form submissions, and responding in real time.
- Building single-page applications (SPAs): In SPAs, pages don’t refresh on each interaction. Instead, content is dynamically swapped out through DOM manipulation.
In web scraping, understanding the DOM is vital as it allows scrapers to target specific elements, like text, buttons, and forms, which can be extracted or automated. For instance, a web scraper can locate and pull data from a table or interact with a search bar by directly accessing the DOM.
How Does DOM Manipulation Work?
DOM manipulation generally follows these steps:
- Select an Element: Identify the element you wish to manipulate. JavaScript provides methods like
getElementById
,querySelector
, andgetElementsByClassName
to select specific nodes within the DOM. - Modify the Element: Once selected, you can modify the element’s properties, content, and attributes. For example, you can change its text content, style, or event listeners.
- Update the DOM: After the modification, the DOM is updated in real-time to reflect changes on the page.
Here’s a simple JavaScript example to illustrate DOM manipulation:
javascript
document.getElementById("myButton").addEventListener("click", function() {
document.getElementById("content").innerHTML = "Content updated!";
});
In this code, a click event on a button (myButton
) changes the content of an element (content
). This is a basic example, but the principle applies across more complex DOM manipulations in modern web applications.
What is DOM Manipulation in React?
In React, DOM manipulation takes on a more optimized form. React uses a concept called the Virtual DOM to handle updates efficiently. Instead of directly interacting with the browser’s DOM, React maintains a virtual representation of the DOM, which is a lightweight copy.
When a change occurs in a React component, React first updates the virtual DOM, compares it with the previous version, and identifies the differences. This approach is known as reconciliation. React then updates only the elements in the actual DOM that have changed, minimizing the amount of DOM manipulation and, as a result, improving performance.
React’s virtual DOM process makes it particularly suitable for applications with a high level of user interaction, as it reduces the cost and complexity of constantly updating the actual DOM.
Here’s a breakdown of how DOM manipulation works in React:
- Component Rendering: When a component’s state or props change, React creates a new virtual DOM representation of that component.
- Reconciliation: React compares this new virtual DOM with the old one, identifying the elements that need updating.
- DOM Updating: React applies only the necessary changes to the actual DOM, ensuring a more efficient update process.
With this approach, React developers don’t need to manipulate the DOM directly. Instead, they update the component’s state or props, and React handles the DOM updates in the background. This abstraction simplifies the development process and significantly boosts performance, especially in complex, data-driven applications.
What is the Difference Between BOM and DOM?
While DOM represents the HTML document, BOM (Browser Object Model) represents the browser’s environment, providing methods to interact with the browser itself rather than the content of a page.
Some key distinctions include:
-
DOM: Focuses on the structure and content of the web page. It provides methods for selecting, creating, and modifying HTML elements, allowing developers to change the visible content of a page.
-
BOM: Focuses on browser-level interactions, like manipulating the window size, navigating to different URLs, or handling browser-specific events. BOM methods include
window.alert()
,window.open()
, andnavigator
.
The BOM acts as the interface between JavaScript and the browser, giving access to features like:
- Window operations: Opening, closing, resizing, and moving the browser window.
- Navigator properties: Information about the browser and operating system, like the user agent.
- History manipulation: Accessing the browser’s history stack to enable back, forward, and go-to actions.
In web development, DOM and BOM work together to provide a full suite of tools for interacting with both the document and the browser environment. While DOM manipulation is primarily concerned with content, BOM provides functionalities that enhance control over the browser, making it crucial for tasks such as managing session states, cookies, and page redirects.
Common Tools for DOM Manipulation
JavaScript libraries and frameworks provide developers with powerful tools for DOM manipulation:
-
jQuery: A well-known library that simplifies DOM manipulation with a more concise syntax. While less common in modern development, it remains useful for quick and straightforward manipulation.
-
React: As discussed, React’s Virtual DOM provides an efficient solution for DOM manipulation in data-driven applications, minimizing direct interactions with the actual DOM.
-
Vue.js: Similar to React, Vue uses a virtual DOM to enhance performance and streamline the manipulation of elements in complex applications.
-
Scrapeless: For web scraping, understanding and manipulating the DOM is crucial. Tools like Scrapeless allow scrapers to interact with the DOM to extract specific data efficiently.
Conclusion
DOM manipulation is a cornerstone of modern web development, enabling developers to create dynamic, interactive, and responsive web applications. Whether through direct manipulation with JavaScript, efficient handling with frameworks like React, or through web scraping with tools that rely on precise DOM interactions, mastering DOM manipulation offers powerful capabilities for managing web content and enhancing user experience.
Understanding the differences between DOM and BOM further adds to a developer's toolkit, providing insights into how to handle both page content and browser-specific features effectively. Together, these elements offer a full spectrum of tools for building, modifying, and controlling the user’s interaction with a webpage, forming the foundation of both modern web development and web scraping practices.
At Scrapeless, we only access publicly available data while strictly complying with applicable laws, regulations, and website privacy policies. The content in this blog is for demonstration purposes only and does not involve any illegal or infringing activities. We make no guarantees and disclaim all liability for the use of information from this blog or third-party links. Before engaging in any scraping activities, consult your legal advisor and review the target website's terms of service or obtain the necessary permissions.