What is a Headless Browser and What is it Used For?
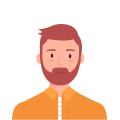
Senior Web Scraping Engineer
A headless browser is a web browser without a graphical user interface (GUI). Unlike standard browsers that show web pages visually, headless browsers work behind the scenes, processing web content without rendering it on a screen. They’re popular tools for automated tasks like web scraping, website testing, and data extraction, where displaying content isn’t necessary. Headless browsers offer a powerful way to interact with web pages programmatically, simulating user interactions to automate various tasks.
What is a Headless Browser and What is it Used For?
A headless browser is a web browser that runs without a graphical user interface (GUI). It can perform all actions a regular browser does—such as navigating web pages, clicking buttons, and filling out forms—but operates in an invisible mode, making it ideal for automated tasks and backend processes that don’t require visual confirmation. This characteristic makes headless browsers highly efficient for data-intensive tasks such as web scraping, automated testing, data extraction, and more.
Common Uses of Headless Browsers
Headless browsers are versatile tools widely used in various areas, including:
-
Web Scraping: Headless browsers can load web pages, run JavaScript, and retrieve content just like a regular browser. This is especially helpful for collecting data from dynamic websites that use JavaScript to render content, where traditional HTTP requests might fall short.
-
Automated Testing: Essential for software development, headless browsers allow developers to automate interactions like clicks, form submissions, and page navigation. This is particularly useful for testing web applications across different environments to ensure all elements work as expected.
-
Data Extraction and Monitoring: Headless browsers can be set up to monitor specific data points on web pages, such as prices, stock availability, or news updates. They’re ideal for real-time data extraction tasks where information frequently changes.
-
Web Performance Analysis: Developers often use headless browsers to assess page load times, rendering speed, and other performance metrics, which can help optimize website performance and user experience.
-
SEO Testing: A headless browser can simulate a search engine crawler's experience, allowing developers to see how their content appears to crawlers and to identify areas for SEO improvement.
Scrapeless' Web Scraping API and Web Unblocker now feature a Headless Browser capability, designed to facilitate the extraction of public data from complex websites. This tool allows users to:
- Set browser instructions to automate interactions
- Adjust browser settings to mimic natural user behavior
- Execute JavaScript to dynamically load additional data
- Try it free today!
What are the Popular Headless Browsers
Here’s an overview of some popular headless browsers, along with their main features and key applications. Each browser has its unique strengths, making it suited for specific web automation tasks:
1. Mozilla Firefox
Mozilla Firefox introduced headless mode in version 56, allowing it to run without a graphical interface. This is a popular choice for web scraping and automated testing due to its open-source nature and strong community support.
Key Features | Use Cases |
---|---|
Multi-platform support, built-in WebDriver, strong security | Web scraping, automated testing, cross-browser testing |
2. HtmlUnit
HtmlUnit is a lightweight headless browser written in Java, mainly used in automated testing environments. It’s minimalistic and does not fully support JavaScript rendering, so it's more suitable for simpler tasks.
Key Features | Use Cases |
---|---|
Java-based, limited JavaScript support, lightweight | Basic automation, simple data extraction |
3. PhantomJS
PhantomJS was one of the earliest popular headless browsers, known for its speed and the ability to render pages completely. However, it's no longer maintained, so it’s used less frequently in new projects.
Key Features | Use Cases |
---|---|
Screenshot support, flexible customizations, quick rendering | Older automation setups, legacy testing |
4. Headless Chrome
Headless Chrome has become the preferred headless browser for many, thanks to full JavaScript and CSS support and access to Chrome’s DevTools. It is highly effective for complex tasks and is widely used in web scraping, testing, and SEO analysis.
Key Features | Use Cases |
---|---|
Full rendering, extensive JavaScript support, DevTools, WebDriver support | Web scraping, SEO analysis, cross-browser testing |
Comparison Table
Headless Browser | JavaScript Support | Maintained | Notable Use Cases |
---|---|---|---|
Mozilla Firefox | Full | Yes | Web scraping, cross-browser testing |
HtmlUnit | Limited | Yes | Simple data extraction |
PhantomJS | Full (limited support) | No | Legacy automation, testing |
Headless Chrome | Full | Yes | SEO analysis, testing, scraping |
Each of these options has a unique focus. Headless Chrome and Firefox are best for complex interactions due to their JavaScript support, while HtmlUnit is ideal for lightweight automation without complex rendering requirements. PhantomJS, although no longer updated, can still serve in some older setups.
What is Headless Browser Testing?
For a long time, developers have relied on UI-driven testing to verify that their applications function correctly. However, this type of testing often encounters issues that impact its effectiveness. One major challenge is stability—UI-driven testing can sometimes fail to interact consistently with the browser, leading to unreliable test results. Another common drawback is the slower speed, as loading and rendering the user interface in a standard browser is resource-intensive and time-consuming.
Headless browser testing offers a solution to these problems. By running tests without loading the browser’s graphical interface, headless testing allows for direct interactions with the webpage, improving both reliability and speed. Tests execute faster, as there’s no overhead from visual rendering, and the direct page interactions make the testing process more stable and efficient. This streamlined approach results in faster, more dependable end-to-end testing for web applications.
Frameworks for Headless Browser Testing
Headless browser testing is often performed using specialized frameworks that automate and streamline the testing process. Several popular frameworks are used to execute headless tests, each offering different features and functionality. Below are some of the most commonly used frameworks for headless browser testing, along with brief descriptions and sample code snippets.
1. Selenium
Selenium is one of the most widely used frameworks for web application testing. It supports multiple browsers, including headless options like Chrome and Firefox, making it suitable for both UI-driven and headless tests.
Example Code (Python):
python
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
options = Options()
options.add_argument("--headless") # Runs Chrome in headless mode
driver = webdriver.Chrome(options=options)
driver.get("http://example.com")
print(driver.title)
driver.quit()
2. Playwright
Playwright is a newer framework that supports headless testing for multiple browsers, including Chromium, Firefox, and WebKit. It's known for its speed and reliability in automating end-to-end tests, particularly for modern web applications.
Example Code (JavaScript):
javascript
const { chromium } = require('playwright'); // Or use 'firefox' or 'webkit'
(async () => {
const browser = await chromium.launch({ headless: true });
const page = await browser.newPage();
await page.goto('http://example.com');
console.log(await page.title());
await browser.close();
})();
3. Puppeteer
Puppeteer is a popular framework for automating Chrome and Chromium browsers. It’s often used for scraping, testing, and rendering dynamic web pages, offering a simple API for headless browser interactions.
Example Code (JavaScript):
javascript
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch({ headless: true });
const page = await browser.newPage();
await page.goto('http://example.com');
console.log(await page.title());
await browser.close();
})();
4. Cypress
Cypress is an end-to-end testing framework designed for web applications. While primarily designed for UI testing, it also supports headless mode for faster execution in continuous integration (CI) environments.
Example Code (JavaScript):
javascript
describe('Headless Test', function() {
it('Visits the example page', function() {
cy.visit('http://example.com');
cy.title().should('include', 'Example Domain');
});
});
5. NightwatchJS
NightwatchJS is an easy-to-use framework for end-to-end testing that integrates well with Selenium WebDriver and supports headless browser testing. It allows for writing tests in JavaScript and has a rich set of APIs for browser interactions.
Example Code (JavaScript):
javascript
module.exports = {
'Demo test Google': function (browser) {
browser
.url('http://example.com')
.waitForElementVisible('body', 1000)
.assert.titleContains('Example Domain')
.end();
}
};
6. PhantomJS
PhantomJS is a headless WebKit browser that provides a robust API for automating web tasks, including scraping, testing, and rendering. However, PhantomJS has been officially discontinued, with modern alternatives like Puppeteer and Playwright now recommended for headless testing.
Example Code (JavaScript):
javascript
var page = require('webpage').create();
page.open('http://example.com', function(status) {
console.log(page.title);
phantom.exit();
});
Limitations of Headless Browser Testing
Headless browser testing provides speed and efficiency but also comes with several limitations. One major issue is the limited visibility into potential layout or UI-related problems. Since the graphical user interface (GUI) is absent, headless browsers do not display the website’s visual aspects, making it challenging to detect issues like broken layouts, misaligned elements, or visual glitches that users may encounter. This limitation can lead to tests passing in headless mode while failing when viewed in a regular browser, resulting in misleading results when testing the user experience of a web application.
Another limitation of headless browser testing is handling certain browser-specific features and events. For example, headless browsers might struggle with JavaScript-heavy applications, especially those that involve animations, media playback, or complex transitions. Additionally, elements that require user interactions, like hovering or dragging, can be harder to simulate in headless mode, which may lead to incomplete test coverage. Despite their ability to execute JavaScript, headless browsers may occasionally differ in behavior compared to full browsers, especially in rendering complex CSS or animations.
For scenarios requiring full rendering or the ability to deal with challenging anti-bot measures, services like Scrapeless offer solid solutions that leverage headless browsers while also handling complex, interactive elements effectively.
Conclusion
Headless browsers are essential tools for modern web development and testing, providing efficient, resource-saving solutions for tasks that don’t need a graphical interface. They’re ideal for automated testing, web scraping, and various backend processes. With a range of frameworks available, such as Selenium, Puppeteer, and Playwright, developers have numerous options for integrating headless browser functionality into their workflows.
At Scrapeless, we only access publicly available data while strictly complying with applicable laws, regulations, and website privacy policies. The content in this blog is for demonstration purposes only and does not involve any illegal or infringing activities. We make no guarantees and disclaim all liability for the use of information from this blog or third-party links. Before engaging in any scraping activities, consult your legal advisor and review the target website's terms of service or obtain the necessary permissions.