What Is Data Parsing? A Beginner’s Guide with Tips and Examples
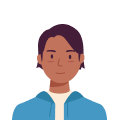
Expert Network Defense Engineer
Each day, around 2.5 quintillion bytes of data are generated globally. This surge in information makes data parsing an essential tool for managing and analyzing data effectively. Simply put, data parsing is the process of extracting specific information from a data source and transforming it into a structured, usable format. This skill is particularly valuable for those handling large datasets.
With data parsing, users can efficiently sift through extensive data, pinpointing the most relevant information and gaining valuable insights that can guide better decision-making. In this blog, we’ll break down the core aspects of data parsing, discuss how it works, and provide examples and tips to help you leverage data parsing for more informed choices.
What Is Data Parsing?
Data parsing refers to the process of converting raw, unstructured data into a structured format. For example, when scraping data from a website, an HTML page may contain a lot of irrelevant elements, such as advertisements or navigation bars. Parsing allows you to target specific sections—like product titles, prices, or descriptions—filtering out unneeded parts. This makes the data easier to work with and analyze in applications or data processing systems.
Consider the following example of parsing HTML content to extract specific data:
python
from bs4 import BeautifulSoup
html_content = '''
<html>
<body>
<h2 class="title">Product A</h2>
<p class="price">$20</p>
</body>
</html>
'''
# Parse HTML content using BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
# Extract title and price
title = soup.find('h2', class_='title').text
price = soup.find('p', class_='price').text
print(f"Title: {title}, Price: {price}")
In this example, we use BeautifulSoup to parse HTML and extract a product title and price, showing how parsing simplifies data extraction.
Popular Data Parsing Techniques and How They Work
Let’s look at several widely used data parsing techniques, each with its unique approach to handling specific data formats.
1. HTML Parsing
HTML parsing is particularly important for web scraping, as HTML is the standard language for web pages. HTML documents are structured in tags (<div>
, <h1>
, <p>
, etc.), each defining different elements.
-
HTML Parsers: Libraries like BeautifulSoup in Python and Cheerio in JavaScript offer functionality to navigate and extract data from HTML by traversing the Document Object Model (DOM).
-
How It Works: Parsers like BeautifulSoup allow you to access elements using CSS selectors, tag names, and class names, making it easy to target specific parts of the document.
Example with BeautifulSoup:
python
from bs4 import BeautifulSoup
import requests
# Request HTML content of a webpage
url = 'https://example.com/products'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
# Extract product names from the webpage
products = soup.find_all('h2', class_='product-name')
for product in products:
print(product.text)
In this code, we retrieve a list of product names by specifying the class name of the <h2>
elements containing each product title. This is a basic yet powerful way to parse and retrieve targeted data from HTML.
2. JSON Parsing
JSON (JavaScript Object Notation) is a popular data format, especially for API responses, as it is lightweight and easy to read for both humans and machines. JSON is commonly used to exchange data between web clients and servers.
- JSON Parsers: Most programming languages provide built-in libraries to parse JSON. For instance, Python has a built-in
json
module, and JavaScript offersJSON.parse
for easy JSON handling. - How It Works: JSON parsers convert JSON data into dictionaries or objects, where each key-value pair can be accessed by its key.
Example in Python:
python
import json
# Sample JSON data (e.g., from an API)
json_data = '{"name": "Alice", "age": 30, "city": "New York"}'
# Parse JSON string into a dictionary
data = json.loads(json_data)
print(f"Name: {data['name']}, Age: {data['age']}, City: {data['city']}")
In this example, the json.loads()
method converts a JSON string into a Python dictionary, making it easier to access specific data points.
3. XML Parsing
XML (Extensible Markup Language) is commonly used for structured data storage and exchange, particularly in older APIs and configuration files. XML data is structured similarly to HTML, with nested tags.
- XML Parsers: Libraries like
xml.etree.ElementTree
in Python or Java’sjavax.xml.parsers
package facilitate XML parsing. - How It Works: XML parsing is similar to HTML parsing; parsers navigate through nodes, retrieving specific elements by tag names or attributes.
Example in Python:
python
import xml.etree.ElementTree as ET
# Sample XML data
xml_data = '''
<user>
<name>Alice</name>
<age>30</age>
<city>New York</city>
</user>
'''
# Parse XML data
root = ET.fromstring(xml_data)
# Extract data
name = root.find('name').text
age = root.find('age').text
city = root.find('city').text
print(f"Name: {name}, Age: {age}, City: {city}")
In this example, we use the ElementTree
library to parse XML data, accessing each piece of information by its tag name.
4. CSV Parsing
CSV (Comma-Separated Values) is a popular format for tabular data, such as spreadsheets. Parsing CSV data is essential in fields like data analysis and reporting.
- CSV Parsers: Python’s
csv
module and data manipulation libraries like Pandas simplify the process of loading and manipulating CSV data. - How It Works: CSV parsers convert each row into a list or dictionary, making it easy to manipulate each record individually.
Example in Python using Pandas:
python
import pandas as pd
# Load CSV data
df = pd.read_csv('data.csv')
# Print first five rows
print(df.head())
# Access specific column
print(df['Product Name'])
With Pandas, parsing CSV files becomes incredibly efficient, allowing for complex data transformations and computations directly on the data.
Tips for Efficient Data Parsing
Parsing can be computationally intensive, especially with large or complex datasets. Here are a few tips to optimize the parsing process:
Absolutely! Here’s a more streamlined and varied version:
1. Choose Tools and Methods Based on Data Structure
The first step toward efficient data parsing is to match your tools and methods to the specific data structure at hand. For HTML, libraries like BeautifulSoup or Cheerio provide straightforward ways to navigate and extract tags, while JSON parsing can be handled effectively with built-in methods in Python or JavaScript. Similarly, for CSV files, using data-handling libraries like Pandas in Python can speed up parsing and allow you to quickly filter, sort, and analyze data. Choosing the right tool for each data structure will make your parsing workflow smoother and more targeted.
2. Batch Processing for Large Files
When dealing with extensive datasets, memory overload can become an issue, leading to crashes or sluggish performance. Instead of parsing the entire file at once, process the data in manageable chunks. Most data libraries, including Pandas, support batch loading, which divides large files into smaller segments, allowing for smoother and faster processing without sacrificing memory. For example, in Python, the chunksize
parameter in Pandas lets you read a large CSV in parts, making it easier to handle millions of rows without lag.
3. Validate and Clean Data Before Parsing
A critical part of parsing is ensuring that data is accurate and in a consistent format. Irregularities—such as unexpected characters, missing fields, or mixed formats—can lead to parsing errors that waste time and yield inaccurate results. Clean the data before parsing by standardizing formats, removing unnecessary characters, and filling in or dropping incomplete values as necessary. Setting up validation checks, such as ensuring numeric columns only contain numbers, helps catch issues early and ensures you’re working with reliable data from the start.
4. Optimize Parsing Speed with Multithreading
For larger datasets, parsing can be time-consuming, especially when running on a single thread. Multithreading allows multiple segments of data to be processed simultaneously, substantially speeding up the parsing process. Python’s multiprocessing
library, for example, enables easy implementation of multithreading, allowing you to handle multiple parsing tasks at once. By leveraging multithreading, you can achieve faster processing times, especially for heavy data parsing tasks, and complete your projects more efficiently.
By focusing on these core strategies—selecting the right tools, processing large files in batches, validating and cleaning data, and leveraging multithreading—you’ll set a strong foundation for efficient, accurate data parsing. These tips not only streamline your workflow but also help ensure the quality and usability of your parsed data.
What are Challenges in Data Parsing
Data parsing can be a complex endeavor, often fraught with various challenges that can hinder efficiency and accuracy. One of the primary difficulties lies in dealing with inconsistent data formats. When data originates from different sources, it can come in a variety of formats—HTML, JSON, XML, CSV—each with its unique structure and idiosyncrasies. This inconsistency necessitates a flexible parsing approach that can adapt to different formats, which can complicate the overall parsing workflow and introduce the risk of errors.
Another significant challenge is managing large datasets. As the volume of data increases, so does the potential for memory overload, slow processing times, and data loss. Parsing massive files all at once can strain system resources, leading to crashes or incomplete operations. This issue can be exacerbated when the data is not properly indexed or organized, making it difficult to efficiently access the required information.
Moreover, data quality is a persistent challenge in parsing. Raw data often contains inaccuracies, such as missing fields, duplicates, or irrelevant information. Cleaning and validating this data before parsing is essential but can be time-consuming. Without thorough preprocessing, the risk of encountering parsing errors increases, which can derail the entire data extraction process.
Finally, dynamic content and anti-scraping measures pose significant hurdles, especially when extracting data from websites. Many websites employ techniques to block automated data extraction attempts, such as CAPTCHAs, IP blocking, and dynamic loading of content. These obstacles not only complicate the parsing process but also require developers to continuously adapt their strategies to circumvent these challenges.
Having trouble with web scraping challenges and constant blocks on the projects you are working on? I use Scrapeless to make data extraction easy and efficient, all in one powerful tool. Try it free today!
Popular Data Parsing Tools
A range of specialized libraries and tools make data parsing easier. Here are a few popular ones:
- BeautifulSoup (Python): Perfect for HTML parsing.
- Cheerio (JavaScript): Ideal for HTML parsing in Node.js.
- Pandas (Python): Great for working with CSV and tabular data.
- json (Python): Built-in library for JSON parsing.
- xml.etree.ElementTree (Python): Useful for XML parsing.
These tools offer various functions for specific parsing needs, enhancing speed and accuracy.
Conclusion
As the volume of data generated daily continues to rise, the significance of data parsing grows correspondingly. For industries and organizations alike, leveraging data parsing is crucial for making informed decisions and extracting valuable insights from the data at hand. While challenges in data parsing are inevitable, employing the right tools, strategies, and methodologies can turn these obstacles into opportunities, ultimately enhancing your business operations.
Frequently Asked Questions (FAQs)
-
How can I determine the best data parsing method for my needs?
To choose the best data parsing method, consider the type of data you are working with, its format (e.g., HTML, JSON, XML), and the specific use case. Analyze your data structure and evaluate various parsing libraries or tools that align with your requirements for efficiency and accuracy. -
Can data parsing be automated?
Yes, data parsing can be automated using scripts or tools that run parsing tasks without manual intervention. Many programming languages offer libraries that allow you to schedule and automate data extraction processes, making it easier to handle recurring tasks. -
What role does data cleaning play in parsing?
Data cleaning is essential in the parsing process as it ensures that the raw data is accurate, consistent, and free of errors. Cleaning data before parsing helps to minimize parsing errors and enhances the reliability of the parsed data for analysis. -
Are there any legal considerations when parsing data from websites?
Yes, there are legal considerations to keep in mind when parsing data, particularly from websites. Always check the site's terms of service to ensure compliance with their data usage policies. Respect robots.txt files and consider copyright laws that may apply to the data you are extracting. -
What are some best practices for handling sensitive data during parsing?
When dealing with sensitive data, ensure that you implement data encryption, limit access to authorized personnel, and comply with data protection regulations such as GDPR. Always anonymize personally identifiable information (PII) when possible and ensure that your parsing tools are secure.
At Scrapeless, we only access publicly available data while strictly complying with applicable laws, regulations, and website privacy policies. The content in this blog is for demonstration purposes only and does not involve any illegal or infringing activities. We make no guarantees and disclaim all liability for the use of information from this blog or third-party links. Before engaging in any scraping activities, consult your legal advisor and review the target website's terms of service or obtain the necessary permissions.