How to Use BeautifulSoup for Web Scraping in Python
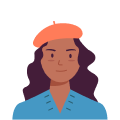
Specialist in Anti-Bot Strategies
Web scraping is a powerful way to collect data from websites and use it for analysis, automation, or just about any data-driven task you can imagine. Python’s BeautifulSoup library, combined with requests, makes it easy and intuitive to scrape web data. In this guide, we’ll cover everything you need to know about using BeautifulSoup for web scraping, from setup to advanced techniques, with detailed code examples along the way.
What is BeautifulSoup?
BeautifulSoup is a Python library designed for web scraping, specifically for parsing HTML and XML documents. It creates a parse tree from page source code, enabling us to interact with and manipulate the content, making it a go-to tool for data extraction. BeautifulSoup is often paired with requests
to fetch webpage content before parsing it.
How Does BeautifulSoup Work?
BeautifulSoup uses parsers to transform HTML or XML documents into a tree structure that can be easily searched and modified. For example, with BeautifulSoup, you can:
- Parse HTML Content: Load the page content into BeautifulSoup using a parser like
html.parser
. - Traverse the DOM: BeautifulSoup’s methods let you access specific elements, attributes, and text within the HTML.
- Extract and Modify Data: Once you locate the target data, you can extract it, modify it, or perform additional actions.
This makes BeautifulSoup ideal for tasks like extracting product information, web data, or automating repetitive actions on a page.
Comparing BeautifulSoup with Other Python Libraries
Several Python libraries can perform web scraping, each with unique strengths. Let’s look at how BeautifulSoup compares with other popular options:
BeautifulSoup vs. Scrapy
Feature | BeautifulSoup | Scrapy |
---|---|---|
Best For | Simple scraping tasks, HTML parsing | Large-scale scraping projects |
Learning Curve | Low, beginner-friendly | Moderate, requires some setup |
Data Extraction | Straightforward, great for small projects | Designed for data extraction pipelines |
Performance | Slower, not optimized for speed | Faster, asynchronous scraping |
Built-in Crawling | No | Yes (built-in crawling and scheduling capabilities) |
Built-in Middleware | No | Yes, allows extensive customization and automation |
Key Takeaway: BeautifulSoup is ideal for small to medium-scale projects and learning web scraping basics, while Scrapy is built for high-performance, large-scale scraping with additional customization options.
BeautifulSoup vs. Selenium
Feature | BeautifulSoup | Selenium |
---|---|---|
Best For | Static HTML scraping | JavaScript-heavy websites |
Interactivity | Limited, cannot interact with elements | Full browser automation |
Performance | Faster, as it only parses HTML | Slower, requires running a browser instance |
Ideal Use Case | Static content scraping | Sites with dynamic, JavaScript-rendered content |
Learning Curve | Low | Moderate |
Key Takeaway: BeautifulSoup is a great choice for static sites, while Selenium is necessary for sites with JavaScript-rendered content, where dynamic interactions (e.g., clicking buttons) are needed.
BeautifulSoup vs. lxml
Feature | BeautifulSoup | lxml |
---|---|---|
Best For | Simple HTML/XML parsing | High-performance XML parsing |
Parsing Speed | Moderate | Very fast |
Parser Flexibility | Compatible with multiple parsers | Focuses on lxml parser, which is faster but limited |
Error Handling | Robust error handling, ideal for poorly formatted HTML | Less forgiving with malformed HTML |
Syntax | Simple and readable | Requires slightly more complex syntax |
Key Takeaway: For XML parsing and speed-critical tasks, lxml
outperforms BeautifulSoup. However, for standard web scraping with HTML, BeautifulSoup offers a simpler, more readable syntax.
When to Use BeautifulSoup
BeautifulSoup is best suited for tasks where:
- The webpage structure is relatively simple and static (i.e., no heavy JavaScript rendering).
- Data is readily accessible in the HTML source, without significant interactivity or dynamic loading.
- Speed isn’t the primary concern, and the focus is on ease of use and flexibility.
For projects that require large-scale scraping or have complex requirements, you may want to explore more advanced solutions like Scrapy or Selenium.
Choosing the Right Parser in BeautifulSoup
BeautifulSoup can parse HTML using different parsers, each with pros and cons:
html.parser
: Python’s built-in HTML parser, which is easy to use and available by default. It’s slower than other parsers but sufficient for most BeautifulSoup projects.lxml
: Fast and reliable,lxml
is ideal for speed-critical tasks. It’s a good choice if you’re dealing with larger datasets and need quick parsing.html5lib
: This parser handles complex HTML5 and poorly formatted HTML exceptionally well, but it’s slower. Use it if you need maximum accuracy with HTML5.
Example: Specifying a parser when creating a BeautifulSoup object:
python
from bs4 import BeautifulSoup
html_content = "<html><body><h1>Hello, World!</h1></body></html>"
soup = BeautifulSoup(html_content, 'lxml') # Using lxml parser for speed
Why Choose BeautifulSoup for Web Scraping?
BeautifulSoup is a lightweight, straightforward option for HTML parsing, making it ideal for both beginners and developers needing quick data extraction. Here are some reasons to choose BeautifulSoup:
- Beginner-Friendly: With simple, readable syntax, BeautifulSoup allows users to focus on data extraction without worrying about complex code.
- Versatile and Flexible: BeautifulSoup can parse and search through HTML, making it suitable for various applications like scraping blogs, product reviews, or small datasets.
- Highly Compatible: BeautifulSoup works seamlessly with
requests
, allowing you to fetch and parse data in just a few lines of code.
With its balance of simplicity, power, and ease of use, BeautifulSoup remains a popular choice for web scraping tasks where speed and JavaScript interaction are not priorities. Understanding when and how to use BeautifulSoup effectively is key to mastering web scraping in Python. For tasks beyond BeautifulSoup’s scope, explore other libraries like Scrapy for advanced scraping needs or Selenium for JavaScript-rendered pages.
Setting Up BeautifulSoup for Web Scraping
Before we start, let’s install BeautifulSoup and requests
, another library that helps us download web pages. Open a terminal or command prompt and run:
bash
pip install beautifulsoup4 requests
This installs:
beautifulsoup4
: The BeautifulSoup library itself.requests
: A popular Python library for making HTTP requests.
Fetching Web Pages with requests
To scrape data from a webpage, we first need to fetch the HTML content. The requests
library lets us do this easily. Here’s how it works:
python
import requests
url = 'https://example.com'
response = requests.get(url)
# Check if the request was successful
if response.status_code == 200:
html_content = response.text
print("Page fetched successfully!")
else:
print("Failed to retrieve the page.")
This code sends a GET request to https://example.com
and checks if the request was successful by verifying the HTTP status code.
Parsing HTML with BeautifulSoup
With the HTML content in hand, we can start using BeautifulSoup to parse it.
python
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
print(soup.prettify()) # Print formatted HTML to get a quick overview of the page structure
Using html.parser
, BeautifulSoup processes the HTML document, creating a navigable tree structure.
Navigating and Searching the DOM
To extract specific data from the page, we need to navigate the DOM (Document Object Model) and locate HTML elements.
Accessing Tags and Their Attributes
BeautifulSoup allows easy access to tags and attributes. Here are a few examples:
python
# Get the title tag
title_tag = soup.title
print("Title:", title_tag.string)
# Access an attribute (e.g., href attribute of a link)
first_link = soup.find('a')
print("First link URL:", first_link.get('href'))
Searching the DOM
BeautifulSoup provides various methods to search for elements:
find()
: Finds the first instance of a tag.find_all()
: Finds all instances of a tag.select()
: Selects elements using CSS selectors.
python
# Find the first paragraph tag
first_paragraph = soup.find('p')
print("First paragraph:", first_paragraph.text)
# Find all links
all_links = soup.find_all('a')
for link in all_links:
print("Link:", link.get('href'))
# Use CSS selectors to find elements
important_divs = soup.select('.important')
print("Important divs:", important_divs)
Example with Class and ID Attributes
python
# Find elements with a specific class
items = soup.find_all('div', class_='item')
for item in items:
print("Item:", item.text)
# Find an element with a specific ID
main_content = soup.find(id='main')
print("Main Content:", main_content.text)
Extracting Data from Web Pages
Once you've located the elements, you can extract data from them.
Extracting Text
python
# Extract text from a paragraph
paragraph = soup.find('p')
print("Paragraph text:", paragraph.get_text())
Extracting Links
python
# Extract all links on the page
links = soup.find_all('a', href=True)
for link in links:
print("URL:", link['href'])
Extracting Images
python
# Extract image sources
images = soup.find_all('img', src=True)
for img in images:
print("Image URL:", img['src'])
Advanced Techniques for BeautifulSoup
To make scraping more efficient and effective, here are some advanced BeautifulSoup techniques:
Using Regular Expressions
BeautifulSoup can match tags using regular expressions for more flexible searches.
python
import re
# Find tags starting with 'h' (e.g., h1, h2, h3, etc.)
headings = soup.find_all(re.compile('^h[1-6]$'))
for heading in headings:
print("Heading:", heading.text)
Navigating the Parse Tree
BeautifulSoup’s tree navigation allows movement between parent, sibling, and child nodes:
python
# Access parent, children, and siblings
parent = first_paragraph.parent
print("Parent tag:", parent.name)
next_sibling = first_paragraph.next_sibling
print("Next sibling:", next_sibling)
children = list(parent.children)
print("Children count:", len(children))
Handling Common Web Scraping Challenges
Dealing with JavaScript-Rendered Content
If the content is loaded by JavaScript, BeautifulSoup alone won’t be enough. For such cases, tools like Scrapeless or headless browsers (e.g.,Puppeteer, Playwright) allow scraping dynamic content.
Avoiding IP Blocking
To prevent being blocked while scraping, consider:
- Using Rotating Proxies: Distribute requests across different IPs.
- Adding Delays: Mimic human-like intervals between requests.
Putting It All Together: A Full Web Scraping Example
Let’s walk through a complete example that scrapes a list of articles from a hypothetical blog.
python
import requests
from bs4 import BeautifulSoup
# Step 1: Fetch the webpage
url = 'https://example-blog.com'
response = requests.get(url)
html_content = response.text
# Step 2: Parse the page with BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
# Step 3: Find all articles
articles = soup.find_all('div', class_='article')
# Step 4: Extract and display article details
for article in articles:
title = article.find('h2').text
summary = article.find('p', class_='summary').text
read_more_url = article.find('a', href=True)['href']
print(f"Title: {title}")
print(f"Summary: {summary}")
print(f"Read more: {read_more_url}\n")
In this example:
- We fetch the HTML content from a blog.
- We parse the page with BeautifulSoup.
- We locate each article and extract its title, summary, and link.
Conclusion
BeautifulSoup is an invaluable tool for web scraping with Python, enabling easy access and extraction of data from web pages. With the skills covered in this guide, you’re well-equipped to start scraping static HTML content. For more complex sites, check out tools like Scrapeless to help with scraping dynamic or JavaScript-heavy pages. Happy scraping!
At Scrapeless, we only access publicly available data while strictly complying with applicable laws, regulations, and website privacy policies. The content in this blog is for demonstration purposes only and does not involve any illegal or infringing activities. We make no guarantees and disclaim all liability for the use of information from this blog or third-party links. Before engaging in any scraping activities, consult your legal advisor and review the target website's terms of service or obtain the necessary permissions.