How to Web Scraping With Python – 2024 Guide
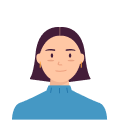
Advanced Data Extraction Specialist
Introduction
In an increasingly digital world, data is more accessible and valuable than ever before. Web scraping, the technique of automatically extracting information from websites, has become an essential skill for developers, researchers, and businesses. Python, with its versatile libraries and straightforward syntax, is a preferred language for web scraping. This guide provides a comprehensive overview of how to use Python for web scraping, highlighting the latest tools, techniques, and best practices in 2024.
Understanding Web Scraping
Web scraping involves fetching and extracting data from web pages. It's used for a wide range of applications, such as market research, price comparison, and academic research. While web scraping is a powerful tool, it's important to consider legal and ethical boundaries. Not all websites allow scraping, and it's crucial to respect the terms of service and privacy policies of the sites you intend to scrape.
Setting Up Your Python Environment
To begin web scraping with Python, you'll need to set up a suitable development environment:
- Python Installation: Ensure you have the latest version of Python installed
- pip: Use pip to install necessary libraries
- Code Editor: Choose a code editor like Visual Studio Code, PyCharm, or Jupyter Notebook for writing and testing your scripts.
Essential Python Libraries for Web Scraping
Several Python libraries are essential for web scraping:
- Requests: A library for making HTTP requests to fetch web pages
- BeautifulSoup: A library for parsing HTML and XML documents
- Selenium: A browser automation tool, useful for interacting with dynamic content
- Scrapy: An advanced web scraping framework for large-scale projects.
Step-by-Step Guide to Web Scraping
1. Fetching Web Pages
The first step in web scraping is to retrieve the web page's HTML content. The requests library is commonly used for this purpose:
language
import requests
url = 'https://example.com'
response = requests.get(url)
html_content = response.text
2. Parsing HTML Content
Once you have the HTML content, you can use BeautifulSoup to parse and navigate the document:
language
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
title = soup.find('title').get_text()
print(title)
This code extracts the title of the page.
3. Handling Dynamic Content
For websites that load content dynamically via JavaScript, Selenium is an effective tool:
language
from selenium import webdriver
driver = webdriver.Chrome()
driver.get('https://example.com')
content = driver.page_source
driver.quit()
This script automates a browser to load the full page content, including dynamically loaded elements.
4. Managing Pagination
To scrape data spread across multiple pages, you'll need to handle pagination. This often involves identifying the pattern in the URLs of subsequent pages and iterating through them.
5. Storing Extracted Data
After extracting the desired data, store it in a format suitable for your needs, such as CSV, JSON, or a database:
language
import pandas as pd
data = {'Title': [title], 'URL': [url]}
df = pd.DataFrame(data)
df.to_csv('data.csv', index=False)
Overcoming Web Scraping Challenges
1. Dealing with Anti-Scraping Measures
Many websites use CAPTCHAs and other anti-scraping technologies to protect their content. Scrapeless, a dedicated web unlocker service, can help navigate these obstacles. By automating CAPTCHA solving and circumventing other security measures, Scrapeless ensures seamless access to data, making it a valuable tool for web scraping.
Fed up with constant web scraping blocks and CAPTCHAs?
Introducing Scrapeless - the ultimate all-in-one web scraping solution!
Unlock the full potential of your data extraction with our powerful suite of tools:
Best CAPTCHA Solver
Automatically solve advanced CAPTCHAs, keeping your scraping seamless and uninterrupted.
Experience the difference - try it for free!
2. Extracting Dynamic Content
Websites that heavily rely on JavaScript for displaying content can be challenging to scrape. While tools like Selenium are helpful, Scrapeless offers a streamlined solution for accessing such content. This service simplifies the process, allowing you to focus on data extraction without worrying about technical hurdles.
3. Data Cleaning and Validation
The data you scrape may require cleaning and validation. Use Python libraries like pandas to preprocess and organize the data, ensuring its quality and consistency.
Best Practices for Ethical Web Scraping
- Respect Website Terms: Always check and adhere to a website’s terms of service
- Use Responsible Request Rates: Avoid overwhelming the server with too many requests
- Implement Error Handling: Gracefully handle HTTP errors and retries
- Respect Privacy: Do not scrape personal data without consent
- Stay Informed: Be aware of the latest legal and ethical guidelines for web scraping.
Conclusion
Web scraping with Python is a powerful way to gather and utilize web data. By following the steps and best practices outlined in this guide, you can scrape data efficiently and ethically. Tools like Scrapeless can help overcome common obstacles, ensuring you have access to the information you need. Whether you are a developer, researcher, or business professional, mastering web scraping can unlock new opportunities and insights.
Start exploring the world of web scraping today, and leverage the power of Python and specialized tools like Scrapeless to access and analyze the web's vast data resources.
At Scrapeless, we only access publicly available data while strictly complying with applicable laws, regulations, and website privacy policies. The content in this blog is for demonstration purposes only and does not involve any illegal or infringing activities. We make no guarantees and disclaim all liability for the use of information from this blog or third-party links. Before engaging in any scraping activities, consult your legal advisor and review the target website's terms of service or obtain the necessary permissions.