What is SSL/TLS Encryption?
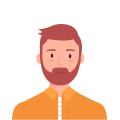
Senior Web Scraping Engineer
SSL (Secure Sockets Layer) and its successor, TLS (Transport Layer Security), are cryptographic protocols that serve as the backbone of secure internet communication. They help protect data exchanged between a client (such as a web browser) and a server (such as a website), ensuring that sensitive information like passwords, credit card numbers, and personal data remain private and unaltered during transmission.
Understanding SSL and TLS
SSL, developed in the mid-1990s by Netscape, was the original protocol that established secure communication over the internet. However, due to various vulnerabilities found in its early versions, SSL was succeeded by TLS in 1999. TLS builds upon SSL's foundation but offers enhanced security and is more efficient. Today, the terms SSL and TLS are often used interchangeably, though TLS is the actual protocol used by modern web browsers.
At its core, SSL/TLS encryption works by creating a secure channel for data transmission. It encrypts data to prevent unauthorized access and eavesdropping by translating readable data into ciphertext, which can only be decrypted by the intended recipient. This process is essential for maintaining data integrity and privacy, particularly when dealing with sensitive information.
Example Code for Establishing an SSL/TLS Connection
To illustrate how SSL/TLS works in practice, here’s a simple Python code snippet that establishes a secure connection to a server using the ssl
module. This example connects to a secure website and retrieves its certificate information.
python
import socket
import ssl
# Define the hostname and port
hostname = 'www.example.com'
port = 443
# Create a socket
sock = socket.create_connection((hostname, port))
# Wrap the socket with SSL
context = ssl.create_default_context()
secure_sock = context.wrap_socket(sock, server_hostname=hostname)
# Retrieve the server's certificate
certificate = secure_sock.getpeercert()
# Display the certificate information
print("Server Certificate:")
for key, value in certificate.items():
print(f"{key}: {value}")
# Close the secure socket
secure_sock.close()
In this code, we create a TCP socket connection to a specified server, wrap it with SSL to establish a secure channel, and then retrieve and print the server's certificate. This example serves as a practical demonstration of how SSL/TLS functions under the hood, allowing secure communication between the client and the server.
What is the Difference Between an SSL and TLS Certificate?
While the terms "SSL certificate" and "TLS certificate" are often used interchangeably, they refer to certificates used to implement the SSL/TLS protocols. The primary difference lies in the protocols they support. An SSL certificate is technically outdated and no longer secure, as SSL has been replaced by TLS. Most modern websites use TLS certificates. However, both types of certificates serve the same purpose: they authenticate the identity of a website and establish a secure, encrypted connection between the web server and the client's browser.
What is SSL Used For?
SSL/TLS encryption is critical for various applications, particularly in securing online communications. Its primary uses include:
-
Securing Websites: SSL/TLS encrypts the connection between a user's web browser and a website, indicated by "HTTPS" in the URL. This ensures that sensitive data such as login credentials and payment information are transmitted securely.
-
Email Protection: Email protocols like SMTPS (Simple Mail Transfer Protocol Secure) and IMAPS (Internet Message Access Protocol Secure) use SSL/TLS to secure email communications, preventing eavesdropping and data breaches.
-
Data Integrity: SSL/TLS ensures that the data exchanged is not tampered with during transmission, maintaining the integrity of the information shared between users and applications.
-
E-commerce Security: Online retailers use SSL/TLS to secure credit card transactions and protect customer information, fostering trust in digital shopping.
Having trouble with web scraping challenges and constant blocks on the project you working?
I use Scrapeless to make data extraction easy and efficient, all in one powerful tool.
Try it free today!
What is SSL/TLS Protection?
SSL/TLS protection refers to the security measures provided by these protocols during data transmission. The main components of SSL/TLS protection include:
-
Encryption: SSL/TLS encrypts the data being transmitted, making it unreadable to anyone who intercepts it. This ensures that sensitive information remains confidential.
-
Authentication: SSL/TLS verifies the identity of the server to which a client connects. This helps prevent attacks where a malicious entity impersonates a legitimate server.
-
Data Integrity: SSL/TLS checks that the data sent between the client and server has not been altered or corrupted during transmission.
Is SSL/TLS Layer 4 or Layer 7?
SSL/TLS operates primarily at the Application Layer (Layer 7) of the OSI model. While it does provide encryption at the transport layer (which could be considered Layer 4), its primary function is to secure application-level data. This means that SSL/TLS is specifically designed to handle protocols like HTTP, SMTP, and others that operate at Layer 7, providing a secure channel for data transmitted between applications.
How SSL/TLS Encryption Works
The process of establishing a secure connection with SSL/TLS involves several key steps:
-
Handshake Process: When a client wants to connect to a secure server, it initiates a process known as the SSL/TLS handshake. This handshake sets up the foundation for secure communication by exchanging cryptographic keys and agreeing on encryption methods.
- Client Hello: The client sends a request to the server, along with information about the encryption algorithms it supports.
- Server Hello: The server responds with its chosen encryption method and a digital certificate, which includes the server’s public key and is verified by a trusted Certificate Authority (CA).
-
Certificate Verification: During the handshake, the client verifies the server's digital certificate against its list of trusted CAs. This step is crucial, as it ensures the server is authentic and that the connection is not being intercepted by an unauthorized entity. If the certificate is valid, the handshake continues.
-
Session Key Creation: Once the server is authenticated, both the client and server create a shared session key. This session key is used to encrypt and decrypt data for the duration of the connection, making it faster than using the server’s public key for each piece of data sent.
-
Data Encryption: With the session key in place, the secure channel is established, and data transmission can begin. Each piece of data sent over the SSL/TLS connection is encrypted before leaving the client and decrypted once it arrives at the server, protecting it from interception and tampering.
-
Secure Termination: When the session ends, the SSL/TLS connection is terminated. Neither the client nor server retains the session key after termination, adding an additional layer of security for future sessions.
Benefits of SSL/TLS Encryption
Implementing SSL/TLS is akin to wrapping your sensitive data in an impenetrable cloak of security, ensuring that only the intended recipient can unveil its contents. This powerful encryption acts as a guardian, shielding your information from prying eyes and malicious third parties who may lurk in the shadows. Beyond safeguarding your data from interception, SSL/TLS also preserves its integrity during transit, ensuring that what you send remains untouched and true to its original form. Furthermore, it serves as a digital bouncer, verifying the identity of the server you’re communicating with and preventing impostors from masquerading as trusted entities. In a world where data breaches are all too common, SSL/TLS stands as a vigilant protector, fostering trust and confidence in online interactions.
Applications and Use Cases of SSL/TLS
SSL/TLS encryption is ubiquitous across the internet and is used by a wide variety of applications. Web browsing is the most common use case, where SSL/TLS secures data exchanges between a user’s browser and a website. Websites that use SSL/TLS encryption are identifiable by “HTTPS” (Hypertext Transfer Protocol Secure) in their URL, indicating that data sent to and from the site is encrypted.
Email communication also heavily relies on SSL/TLS encryption, with protocols like SMTPS (Simple Mail Transfer Protocol Secure) and IMAPS (Internet Message Access Protocol Secure) ensuring that emails remain private. Similarly, SSL/TLS is applied to instant messaging and VoIP (Voice over Internet Protocol) to prevent eavesdropping on private conversations.
E-commerce platforms rely on SSL/TLS to protect payment data, ensuring that credit card details and personal information are not accessible to unauthorized individuals. Online payment processors, including services like PayPal, utilize SSL/TLS to secure transaction data from the user to the payment gateway. Additionally, VPNs (Virtual Private Networks) often use SSL/TLS encryption to secure internet traffic from users to VPN servers, creating a safer browsing experience and concealing user activity from malicious actors.
Evolution and Security Enhancements in SSL/TLS
While SSL/TLS has evolved to meet the demands of modern internet security, vulnerabilities have been discovered over time. For instance, the Heartbleed bug in 2014 exposed a critical flaw in OpenSSL, an implementation of SSL/TLS, which allowed attackers to read sensitive data directly from server memory. As a result, organizations worldwide updated their servers and renewed their SSL/TLS certificates to ensure secure connections.
TLS has also undergone several updates to maintain security. TLS 1.3, released in 2018, is the most current and secure version. It offers improved performance with reduced latency and enhanced encryption methods, which make it resistant to a wide range of cyberattacks. TLS 1.3 also removed outdated cryptographic algorithms, providing a simpler, faster, and more secure experience.
Conclusion
SSL/TLS encryption is a cornerstone of secure internet communication, providing privacy, integrity, and authentication for online data exchanges. From securing website connections to protecting sensitive data in emails, SSL/TLS has become essential in maintaining trust and security in digital interactions. By encrypting data, authenticating servers, and ensuring data integrity, SSL/TLS supports the foundations of modern cybersecurity. As digital threats evolve, the SSL/TLS protocol continues to adapt, underscoring its importance as an indispensable tool for protecting sensitive information across the web.
At Scrapeless, we only access publicly available data while strictly complying with applicable laws, regulations, and website privacy policies. The content in this blog is for demonstration purposes only and does not involve any illegal or infringing activities. We make no guarantees and disclaim all liability for the use of information from this blog or third-party links. Before engaging in any scraping activities, consult your legal advisor and review the target website's terms of service or obtain the necessary permissions.