How to Bypass Rate Limiting When Web Scraping
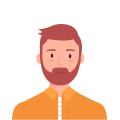
Senior Web Scraping Engineer
Ever started scraping a website, only to find yourself blocked by frustrating rate limits? I’ve been there too. It’s that feeling of finally getting the right data only to be halted by a “429 Too Many Requests” message. But don’t let this stop you. Rate limiting, although designed to control excessive traffic, isn’t the end of your scraping journey. By understanding rate limits and implementing creative solutions, you can still gather the data you need while staying under the radar.
In this guide, we’ll dive into what rate limiting is, why websites use it, and the most effective ways to bypass it while web scraping. Let’s walk through the steps, use a few code examples, and uncover the methods that can make your scraping endeavors smoother.
What is Rate Limiting?
Rate limiting is essentially a strategy used by websites to control the number of requests they allow within a given time frame, often enforced to maintain server health and ensure fair access. Websites typically cap requests to prevent high traffic, protect against abuse, and safeguard resources for all users. For example, a website may limit requests to 100 per minute per user. If your scraper exceeds this limit, the server responds with a “429 Too Many Requests” status code, temporarily blocking further access.
Understanding rate limiting helps to identify how to work around it. Many websites rely on rate limits to balance user access and server load. For more advanced sites, rate limits are combined with CAPTCHAs or IP restrictions, further complicating the process for scrapers.
Why APIs and Websites Use Rate Limiting
Websites and APIs implement rate limiting for several reasons, some of which may impact your scraping goals. Knowing these motives can inform your approach to bypassing rate limits without causing harm or getting blocked entirely.
Preventing Server Overload
Imagine the traffic on a popular site: thousands, if not millions, of users accessing resources simultaneously. Rate limiting prevents any single user—or bot—from monopolizing server resources, which could slow down or even crash the site. It allows servers to handle traffic efficiently by capping request volume. For scrapers, this means that excessive requests might trigger rate limits faster during peak traffic.
Mitigating Abuse
Rate limits also act as a barrier against spammers and malicious bots. When scrapers or bots send numerous requests quickly, rate limits kick in to prevent abuse, maintain security, and deter attacks like denial-of-service (DoS). This can be an issue for scrapers aiming to collect data efficiently, as sites use rate limits to throttle non-human activity.
Encouraging Paid Usage
For APIs, rate limits are often part of a tiered pricing model. Free users may face lower rate limits, while paid subscribers gain access to higher limits or even dedicated API access. This model promotes upgrades by restricting free users while monetizing high-volume data access. Many public APIs like Twitter’s and Google’s use this approach.
Having trouble with web scraping challenges and constant blocks on the project you working?
I use Scrapeless to make data extraction easy and efficient, all in one powerful tool.
Try it free today!
How to Bypass Rate Limiting in Web Scraping
While rate limiting can make scraping challenging, various techniques can help you bypass or minimize its impact effectively. Let’s explore these solutions with code examples and see how you can implement them to avoid being blocked.
1. Using Proxies
Using multiple proxies to distribute requests across different IPs is a classic strategy to bypass rate limits. This approach helps spread traffic across multiple sources, which makes it harder for websites to detect and block your scraper.
python
import requests
from itertools import cycle
# Proxy list
proxies = ["http://proxy1.example.com", "http://proxy2.example.com", "http://proxy3.example.com"]
proxy_pool = cycle(proxies)
url = "https://example.com/data"
for i in range(100):
proxy = next(proxy_pool)
response = requests.get(url, proxies={"http": proxy, "https": proxy})
print(response.status_code)
The code above uses a rotating proxy pattern where each request is sent through a different proxy server. By simulating traffic from multiple locations, you’re less likely to hit rate limits tied to a single IP.
2. Randomizing Delays
Human behavior is often erratic, so adding random delays between requests can mimic real users, making it harder for rate-limit rules to catch on to your patterns. Random delays make your scraper less predictable, which can keep it under the radar.
python
import time
import random
import requests
url = "https://example.com/data"
for i in range(100):
response = requests.get(url)
print(response.status_code)
# Randomized delay between 1 and 5 seconds
time.sleep(random.uniform(1, 5))
By using random.uniform(1, 5)
, you’re introducing natural breaks between requests, reducing the chance of hitting rate limits. This approach works well with websites that allow moderate traffic but enforce strict limits on burst requests.
3. Rotating User Agents
Web servers check headers like the User-Agent to identify the client making the request. By rotating User-Agent strings, your scraper can mimic different browsers, devices, or operating systems, making it appear as though requests come from various users.
python
import requests
import random
url = "https://example.com/data"
user_agents = [
"Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36",
"Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/605.1.15 (KHTML, like Gecko) Version/14.1.1 Safari/605.1.15",
"Mozilla/5.0 (Linux; Android 10) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/90.0.4430.91 Mobile Safari/537.36"
]
for i in range(100):
headers = {"User-Agent": random.choice(user_agents)}
response = requests.get(url, headers=headers)
print(response.status_code)
Rotating User-Agent headers makes it more challenging for servers to detect scraping activity. It’s an effective way to bypass rate limiting by distributing requests across different client profiles.
4. Using Exponential Backoff
When working with APIs, implementing an exponential backoff strategy is a common way to adapt when rate limits are hit. With exponential backoff, you double the delay after each limit hit, reducing the request rate gradually until access is restored.
python
import requests
import time
url = "https://api.example.com/data"
retry_delay = 1
for i in range(100):
response = requests.get(url)
if response.status_code == 429: # Rate limit hit
print("Rate limit reached, backing off...")
time.sleep(retry_delay)
retry_delay *= 2 # Double the delay each time rate limit is hit
else:
print(response.status_code)
retry_delay = 1 # Reset delay after successful request
This technique is commonly used with APIs that enforce strict rate limits. By backing off each time you hit the limit, you can avoid continuous blocking while keeping your scraper active.
5. Managing Session and Cookies
When websites enforce rate limits based on session or cookies, using session management in requests can help simulate persistent user sessions. This approach works well for websites that monitor user behavior over time.
python
import requests
url = "https://example.com/data"
session = requests.Session() # Persistent session
for i in range(100):
response = session.get(url)
print(response.status_code)
Using sessions allows your requests to maintain cookies between calls, which can mimic real user browsing patterns and reduce the likelihood of hitting rate limits.
Conclusion
Bypassing rate limiting is an essential skill in web scraping, especially when gathering data efficiently and staying under detection thresholds. Rate limits are there to protect website resources and provide equal access to all users, but with the right techniques—such as rotating proxies, introducing random delays, managing headers, implementing backoff strategies, and using persistent sessions—scrapers can work around these restrictions responsibly. Remember, effective web scraping involves respecting website policies and finding sustainable solutions that keep both your scraper and the server environment in balance.
At Scrapeless, we only access publicly available data while strictly complying with applicable laws, regulations, and website privacy policies. The content in this blog is for demonstration purposes only and does not involve any illegal or infringing activities. We make no guarantees and disclaim all liability for the use of information from this blog or third-party links. Before engaging in any scraping activities, consult your legal advisor and review the target website's terms of service or obtain the necessary permissions.