Understanding Distributed Architecture for Web Scraping
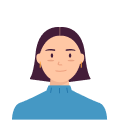
Advanced Data Extraction Specialist
Understanding Distributed Architecture for Efficient Web Scraping
A distributed architecture plays a pivotal role in ensuring efficiency and reliability by utilizing multiple servers to handle various scraping processes. This article delves into the fundamentals of distributed architecture and its advantages in web scraping, focusing on key concepts such as horizontal scaling and fault tolerance.
What is Distributed Architecture?
Distributed architecture refers to a system design that distributes processing and data across multiple nodes, typically across different physical locations. This approach enhances performance, increases redundancy, and improves the overall reliability of web scraping operations. By using a distributed architecture, scraping tasks can be executed concurrently, leading to faster data retrieval and improved resource management.
What is an Example of a Distributed Network Architecture?
A prominent example of a distributed network architecture is Amazon Web Services (AWS). AWS operates a vast network of data centers across the globe, allowing for distributed computing, storage, and data management. Within AWS, services like Amazon EC2 (Elastic Compute Cloud) enable users to deploy applications across multiple virtual servers, ensuring high availability and scalability. Additionally, AWS utilizes load balancers to distribute incoming traffic across these servers, optimizing resource utilization and enhancing fault tolerance.
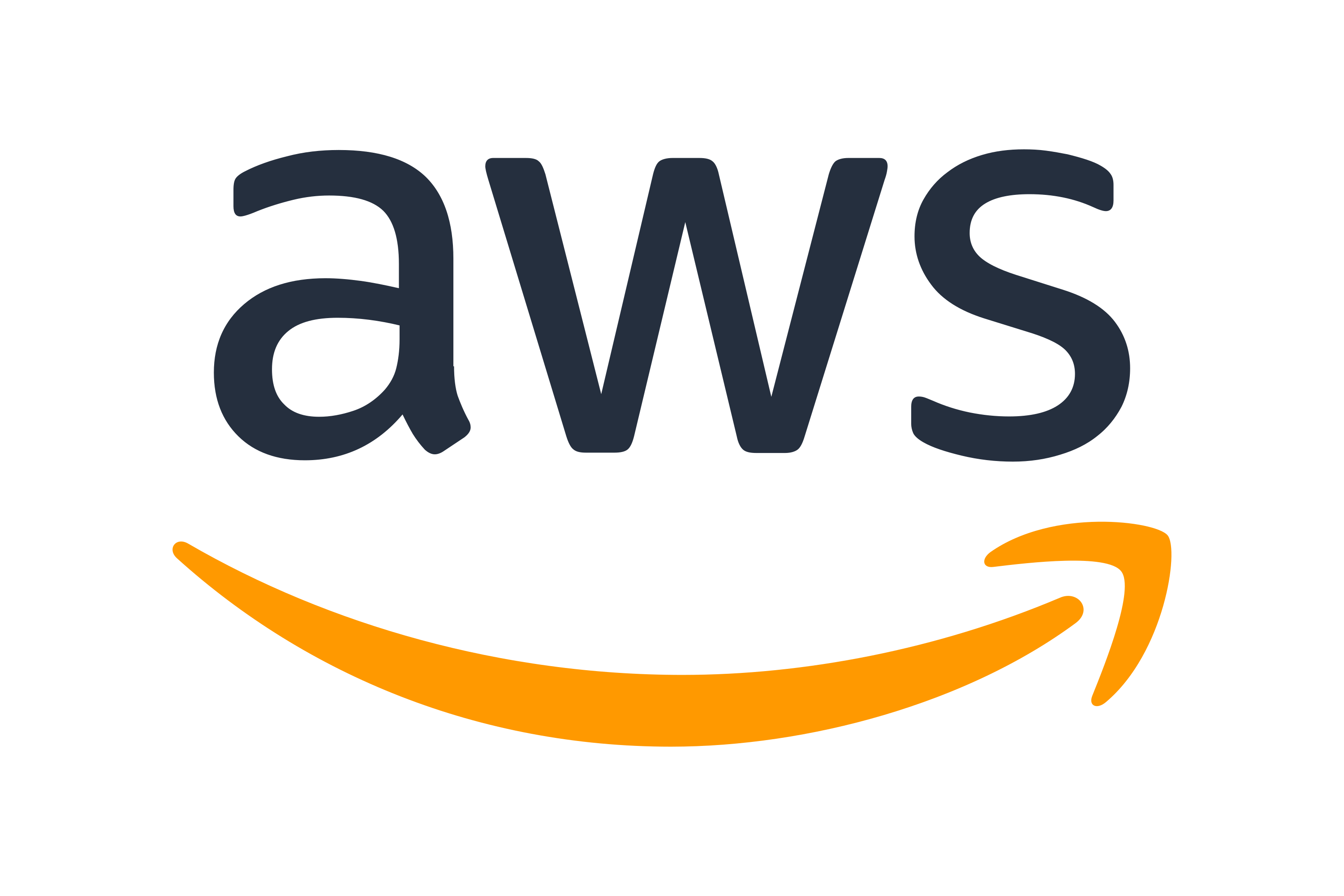
What is the Difference Between Centralized and Distributed Architecture?
-
Centralized Architecture: In a centralized architecture, all processing and data storage occur at a single node or server. This can lead to bottlenecks and single points of failure, making the system less resilient.
-
Distributed Architecture: In contrast, a distributed architecture spreads processing and data across multiple nodes, which can be located in different geographic locations. This enhances performance, redundancy, and reliability, as tasks can continue even if one node fails.
What is Benefits of Distributed Architecture in Web Scraping
1. Horizontal Scaling
Horizontal scaling, or scaling out, involves adding more servers or nodes to a system to distribute the workload effectively. This method is particularly beneficial in web scraping due to the following reasons:
- Increased Throughput: By adding more servers, multiple scraping tasks can be executed simultaneously, significantly increasing the amount of data collected > within a shorter time frame.
- Load Balancing: A distributed system can efficiently balance the load among servers, preventing any single node from becoming a bottleneck.
- Cost Efficiency: Horizontal scaling often leads to reduced costs, as organizations can leverage commodity hardware to build their infrastructure.
If you're looking for a solution that simplifies this process, Scrapeless API offers robust features to manage your scraping tasks efficiently while ensuring high availability and scalability. Also for you can try it for free now
Example of Horizontal Scaling
In this example, we use Python's multiprocessing module to create a simple horizontal scaling solution for scraping multiple URLs concurrently.
python
import requests
from multiprocessing import Pool
# Function to scrape a single URL
def scrape_url(url):
response = requests.get(url)
return response.text[:100] # Return the first 100 characters
# List of URLs to scrape
urls = [
'https://example.com',
'https://example.org',
'https://example.net',
'https://example.edu',
]
# Using Pool to scrape URLs concurrently
if __name__ == '__main__':
with Pool(processes=4) as pool:
results = pool.map(scrape_url, urls)
for result in results:
print(result)
2. Fault Tolerance
Fault tolerance is the ability of a system to continue operating properly in the event of a failure of one or more components. In web scraping, fault tolerance is crucial for ensuring uninterrupted data collection:
- Resilience to Node Failures: A well-designed distributed architecture can detect failed nodes and reroute tasks to functional servers, minimizing downtime.
- Data Redundancy: By replicating data across multiple servers, the risk of data loss is significantly reduced, ensuring that scraping tasks can resume even after a failure.
- Graceful Degradation: In the event of a partial failure, the system can continue to operate at reduced capacity rather than failing completely, allowing for ongoing data collection.
Example of Fault Tolerance
In a distributed system, you can implement a retry mechanism to handle failures. Below is an example using the requests library with a simple retry logic.
python
import requests
from requests.exceptions import RequestException
import time
# Function to scrape a single URL with retries
def scrape_url_with_retry(url, retries=3):
for attempt in range(retries):
try:
response = requests.get(url)
response.raise_for_status() # Raise an error for bad responses
return response.text[:100] # Return the first 100 characters
except RequestException as e:
print(f"Error fetching {url}: {e}. Retrying {attempt + 1}/{retries}...")
time.sleep(2) # Wait before retrying
return None # Return None if all retries fail
# Scraping multiple URLs with fault tolerance
urls = ['https://example.com', 'https://invalid-url']
for url in urls:
result = scrape_url_with_retry(url)
if result:
print(result)
else:
print(f"Failed to fetch {url} after multiple retries.")
Implementing Distributed Architecture for Web Scraping
1. Decentralized Task Management
Implementing a centralized task manager can help coordinate scraping activities across distributed nodes. This manager can queue tasks, distribute them to available servers, and monitor their progress. Tools like Apache Kafka or RabbitMQ are commonly used for message queuing in distributed systems.
2. Data Storage Solutions
Selecting the right data storage solution is vital in a distributed architecture. Options like NoSQL databases (e.g., MongoDB, Cassandra) allow for flexible data models and can scale horizontally, making them suitable for storing large volumes of scraped data.
Example of Using MongoDB for Storage
Here's a simple example of how to save scraped data into a MongoDB database:
python
from pymongo import MongoClient
# Connect to MongoDB
client = MongoClient('mongodb://localhost:27017/')
db = client['web_scraping']
collection = db['scraped_data']
# Function to save scraped data
def save_data(url, content):
document = {'url': url, 'content': content}
collection.insert_one(document)
print(f"Data saved for {url}")
# Example of scraping and saving data
for url in urls:
content = scrape_url(url)
if content:
save_data(url, content)
3. Monitoring and Maintenance
Regular monitoring is essential to ensure the health of each node within the distributed system. Implementing tools like Prometheus or Grafana can provide real-time insights into server performance, helping identify issues before they escalate.
Challenges of Distributed Architecture
While distributed architecture offers numerous advantages, it also presents challenges that must be addressed:
- Network Latency: Communication between nodes can introduce latency, affecting overall performance. Strategies like caching and local processing can help mitigate these issues.
- Complexity in Deployment: Setting up a distributed system can be more complex than a centralized approach, requiring careful planning and coordination among teams.
- Data Consistency: Ensuring consistency across distributed data stores can be challenging. Implementing strong consistency models or eventual consistency strategies is crucial for maintaining data integrity.
- Rate Limiting and IP Management: Many websites impose rate limits or IP restrictions, making it challenging to maintain smooth, uninterrupted scraping operations. A distributed setup, however, allows the use of multiple IP addresses and adaptive request rates to minimize the risk of blocks and delays.
When dealing with rate limits, distributed architectures benefit greatly from solutions like Scrapeless API. Websites often restrict the number of requests allowed within a specific timeframe, resulting in potential delays or temporary IP bans. With Scrapeless API, you can overcome these limitations seamlessly: the service automatically manages IP rotations and distributes requests across multiple servers, allowing for uninterrupted scraping without manual intervention. This automated approach ensures consistent access to data while respecting rate limits, making it ideal for large-scale web scraping tasks that require stable, high-throughput operations.
Conclusion
A distributed architecture is essential for managing large-scale web scraping tasks effectively. Through horizontal scaling and fault tolerance, organizations can improve their data collection capabilities while minimizing downtime and maximizing resource efficiency. As the demand for data continues to grow, embracing a distributed approach will enable more robust and scalable web scraping solutions, allowing businesses to thrive in the data-driven landscape.
By understanding the principles of distributed architecture and incorporating code examples into your workflows, organizations can build a more resilient and efficient web scraping infrastructure that meets their growing data needs. With tools like Scrapeless API, optimizing and managing your scraping tasks becomes even easier.
At Scrapeless, we only access publicly available data while strictly complying with applicable laws, regulations, and website privacy policies. The content in this blog is for demonstration purposes only and does not involve any illegal or infringing activities. We make no guarantees and disclaim all liability for the use of information from this blog or third-party links. Before engaging in any scraping activities, consult your legal advisor and review the target website's terms of service or obtain the necessary permissions.