What is Audio Fingerprinting: A Comprehensive Guide
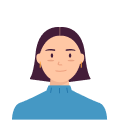
Advanced Data Extraction Specialist
For now, music streaming, broadcasting, and social media are vast and dynamic, audio fingerprinting has become indispensable. Imagine being able to instantly recognize a song played in a coffee shop, or track unauthorized use of copyrighted audio material. These are made possible through audio fingerprinting, a technology that creates unique identifiers (or “fingerprints”) for audio content, enabling quick and accurate recognition even under varying conditions.
In this article, we’ll take a deep dive into what audio fingerprinting is, how it works, and explore practical applications, such as music recognition and copyright management. Additionally, we’ll walk through an implementation in Python, where we’ll create fingerprints using real audio data and demonstrate how to match them effectively. By the end, you’ll have a solid understanding of how to build your own audio fingerprinting solution.
What is Audio Fingerprinting?
Audio fingerprinting is a process that creates a distinctive and condensed representation of an audio sample. Unlike metadata (such as tags and descriptions), an audio fingerprint is based on the unique characteristics within the sound wave itself. Think of it as a “barcode” for audio: a condensed, computational representation that can be matched to a vast library of known “fingerprints.” This allows software to identify the same or similar audio even if it’s altered (e.g., changed in pitch, compressed, or mixed with other sounds).
In essence, audio fingerprinting transforms complex audio data into something like a searchable ID number. This unique fingerprint can then be compared against a database to find matches, enabling applications like music identification apps (e.g., Shazam), broadcast monitoring, and more.
How Audio Fingerprinting Works
The audio fingerprinting process consists of several main steps: preprocessing the audio, generating a spectrogram, extracting distinct features, and creating a unique hash from those features. Let's break down each part of the process to see how a simple audio file is transformed into a digital fingerprint.
Preprocessing the Audio
The first step is to preprocess the audio to prepare it for analysis. This involves:
- Converting stereo to mono (if necessary) to reduce data complexity.
- Resampling to a uniform sample rate to make comparison easier.
- Segmenting the audio to improve efficiency and accuracy.
By standardizing these parameters, we can ensure that the audio is in a consistent format for further processing, which is crucial for accurate fingerprint generation.
Spectrogram Generation
A spectrogram is a graphical representation of audio, mapping time on the x-axis, frequency on the y-axis, and amplitude as color intensity. This visual representation allows us to see the distribution of frequencies in the audio and track how these frequencies change over time. To create a spectrogram in Python, we can use the librosa
library, which provides tools for time-frequency analysis.
Here’s how we generate a spectrogram from an audio file:
python
import numpy as np
import librosa
import librosa.display
import matplotlib.pyplot as plt
# Load the audio file
audio_path = 'sample_audio.wav'
y, sr = librosa.load(audio_path)
# Generate the spectrogram
S = np.abs(librosa.stft(y))
S_db = librosa.amplitude_to_db(S, ref=np.max)
# Display the spectrogram
plt.figure(figsize=(12, 8))
librosa.display.specshow(S_db, sr=sr, x_axis='time', y_axis='log')
plt.colorbar(format="%+2.0f dB")
plt.title('Spectrogram')
plt.show()
In this example, S
represents the magnitude of the audio’s frequencies. We then convert this magnitude to a decibel scale (S_db
), which is more suitable for fingerprinting as it highlights the perceptually important aspects of the audio.
Feature Extraction
Once the spectrogram is generated, the next step is to identify key features within it. Audio fingerprinting relies on identifying unique points—often called anchors—that stand out in the spectrogram. These anchors are typically peaks in amplitude within specific frequency ranges, representing prominent sounds or patterns in the audio.
In Python, we can use maximum_filter
from the scipy.ndimage
library to locate these peaks:
python
from scipy.ndimage import maximum_filter
# Identify peaks in the spectrogram
def extract_peaks(S_db, threshold=10):
peaks = maximum_filter(S_db, size=10) == S_db
rows, cols = np.where(peaks)
peaks_db = [(col, row) for col, row in zip(cols, rows) if S_db[row, col] > threshold]
return peaks_db
peaks = extract_peaks(S_db)
Here, we filter out lower peaks by setting a threshold, which ensures that only the most significant features are selected. This step dramatically reduces the data, capturing only the unique "signature" points necessary for creating the fingerprint.
Creating the Fingerprint Hash
After feature extraction, the unique points (or “anchors”) are hashed to create a compact and searchable representation of the audio file. This hash will act as our audio fingerprint, which can then be stored in a database for future comparison.
A simple method is to combine the coordinates of each peak point into a tuple and hash them. Here’s an example:
python
# Generate a fingerprint by hashing peaks
fingerprint = hash(tuple(peaks))
print(f"Generated fingerprint: {fingerprint}")
This fingerprint is effectively a condensed, high-level representation of the audio sample, which can be stored in a database to facilitate fast matching.
Applications of Audio Fingerprinting
The technology of audio fingerprinting underpins several widely used applications:
- Music Recognition: Applications like Shazam use audio fingerprinting to identify songs. When a user records a short clip, the app generates a fingerprint and checks for a match in its database.
- Copyright Protection: Audio fingerprinting helps identify unauthorized uses of copyrighted content by scanning broadcasts or internet streams for matches.
- Broadcast Monitoring: Radio stations, TV networks, and streaming platforms use fingerprinting to verify that advertisements or specific content are broadcast as required.
- Audio Forensics: Fingerprinting can help identify audio from crime scenes or in legal investigations, matching voice samples to suspects or verifying recordings.
Building a Matching System for Audio Fingerprints
In a real-world setting, we can store audio fingerprints in a database and compare new audio fingerprints against this database to identify matches. Here’s a simple implementation using Python’s sqlite3
to store and retrieve audio fingerprints.
python
import sqlite3
# Connect to the database (or create it)
conn = sqlite3.connect('audio_fingerprints.db')
c = conn.cursor()
# Create a table to store fingerprints
c.execute('''CREATE TABLE IF NOT EXISTS fingerprints (song_name TEXT, fingerprint TEXT)''')
# Add a fingerprint to the database
def add_fingerprint(song_name, fingerprint):
c.execute("INSERT INTO fingerprints (song_name, fingerprint) VALUES (?, ?)", (song_name, fingerprint))
conn.commit()
# Retrieve a match from the database
def match_fingerprint(fingerprint):
c.execute("SELECT song_name FROM fingerprints WHERE fingerprint=?", (fingerprint,))
result = c.fetchone()
return result[0] if result else "No match found"
# Add a sample fingerprint
add_fingerprint("Sample Song", str(fingerprint))
print("Match result:", match_fingerprint(str(fingerprint)))
In this example, we’ve created a basic database structure where each fingerprint is associated with a song name. When we want to identify a new audio sample, we generate its fingerprint and compare it with entries in the database.
Visualizing Peaks on the Spectrogram
For a better understanding of how unique points are chosen, we can overlay the identified peaks onto the spectrogram. This provides a visual representation of the extracted features.
python
# Plot spectrogram with identified peaks
plt.figure(figsize=(12, 8))
librosa.display.specshow(S_db, sr=sr, x_axis='time', y_axis='log')
plt.scatter([p[0] for p in peaks], [p[1] for p in peaks], marker='o', color='r', label='Peaks')
plt.colorbar(format="%+2.0f dB")
plt.title('Spectrogram with Peaks')
plt.legend()
plt.show()
This plot shows the selected peaks over time and frequency, visually indicating the unique characteristics that form the fingerprint.
How to Prevent Audio Fingerprinting
In some cases, particularly in web scraping or automated browsing, preventing audio fingerprinting can be essential for avoiding detection. Audio fingerprinting can be used by websites to identify or track users through their devices' audio configurations, and scrapers may need to simulate or disable audio processing to evade such detection methods.
To prevent audio fingerprinting, scrapers and bots can use several techniques, such as:
- Disabling Audio Processing: Prevent the browser or scraper from processing audio files by disabling audio APIs, thereby minimizing the data available for fingerprinting.
- Simulating Audio Characteristics: Use emulation to simulate a consistent audio environment across sessions, reducing the uniqueness of the audio “fingerprint.”
- Configuring Browser Options: Tools like headless browsers often provide options to disable or modify audio contexts to make fingerprints less identifiable.
By incorporating these measures, scrapers can avoid detection based on audio fingerprints, helping maintain anonymity and stability.
Tip: For effective scraping with minimized detection risk, consider using Scrapeless, providing a headless browser solution with built-in real fingerprinting technology and customizable settings. Scrapeless with them human-like behavior, dynamic page data handling, and the ability to adjust browser features to avoid blocking.
Now You Can Try it with Free
Conclusion
Audio fingerprinting is a powerful technology that enables efficient and accurate audio identification, providing essential support for music recognition apps, copyright enforcement, broadcast monitoring, and more. By extracting unique features from an audio sample, we create a fingerprint that is resilient to alterations and can be rapidly matched against a large database.
Through the provided code examples, you now have a solid foundation for creating and comparing audio fingerprints. This guide can be expanded by incorporating more sophisticated algorithms, such as machine learning for feature extraction or locality-sensitive hashing (LSH) to further enhance fingerprint matching accuracy.
Further Learning
Consider exploring more advanced fingerprinting systems or leveraging libraries like dejavu
for real-time audio matching. Experiment with different types of audio and fingerprinting techniques to gain a deeper understanding of how this technology adapts to various conditions and use cases.
At Scrapeless, we only access publicly available data while strictly complying with applicable laws, regulations, and website privacy policies. The content in this blog is for demonstration purposes only and does not involve any illegal or infringing activities. We make no guarantees and disclaim all liability for the use of information from this blog or third-party links. Before engaging in any scraping activities, consult your legal advisor and review the target website's terms of service or obtain the necessary permissions.