What Is Asynchronous Programming? vs. Synchronous Programming
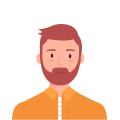
Senior Web Scraping Engineer
Efficiently handling multiple tasks simultaneously has become critical as web applications grow more dynamic and data-driven. Asynchronous programming plays a significant role in modern software design by enabling applications to manage many tasks concurrently without slowing down. By diving into the fundamentals of asynchronous programming, event-driven architecture, and concurrency, developers can improve both the speed and performance of their applications.
In this article, we’ll explore how asynchronous programming works, how it differs from synchronous programming, and how it’s applied in real-world applications. We’ll also look at some practical implementations using async frameworks in popular languages like Python and JavaScript.
What is Asynchronous vs. Synchronous Programming?
Before diving into asynchronous programming, let’s understand how it differs from synchronous programming. Synchronous programming follows a sequential, or “one-at-a-time,” approach. Each task in a synchronous program must wait for the previous task to complete before starting, which can lead to slow performance, especially for I/O-bound tasks like file reading, network requests, or database interactions. Here’s a quick example in Python of a synchronous code flow:
python
import time
def task_one():
time.sleep(2)
return "Task one completed."
def task_two():
time.sleep(2)
return "Task two completed."
# Sequential execution
print(task_one())
print(task_two())
In this case, task_two()
cannot start until task_one()
completes, making the program unnecessarily slow.
Asynchronous programming, on the other hand, allows multiple tasks to run concurrently. Instead of waiting for each task to complete, asynchronous programs initiate tasks and proceed with other work. When a task completes, the program picks up its results, allowing a more efficient workflow. Here’s a look at the asynchronous version of the code using Python’s asyncio
library:
python
import asyncio
async def task_one():
await asyncio.sleep(2)
return "Task one completed."
async def task_two():
await asyncio.sleep(2)
return "Task two completed."
# Concurrent execution with asyncio
async def main():
results = await asyncio.gather(task_one(), task_two())
print(results)
# Run the main event loop
asyncio.run(main())
In this example, task_one
and task_two
run simultaneously, reducing the total runtime and increasing efficiency.
Why is Asynchronous Programming Important?
With modern applications handling massive amounts of data and making multiple simultaneous requests to databases, APIs, and other services, synchronous programming simply doesn’t scale well. Asynchronous programming allows applications to handle high traffic and large data volumes more efficiently, enabling:
- Non-blocking operations: Critical for maintaining fast response times and improving user experience.
- Improved resource usage: Applications can maximize CPU and memory usage by managing multiple tasks concurrently.
- Scalable applications: Asynchronous programming is essential for scaling applications that require rapid data handling, such as web servers, financial software, and real-time systems.
Event-driven Architecture with Async/Await
One of the key components of asynchronous programming is the event-driven architecture. Event-driven systems react to events—like user actions, sensor outputs, or messages—using non-blocking I/O operations. This approach is made simpler and more readable by the async/await
syntax, which allows developers to write asynchronous code in a synchronous style.
In an event-driven architecture, the main program sets up “listeners” that wait for events to occur, and when they do, the program handles them asynchronously. This model is highly efficient for handling multiple events concurrently without creating delays.
Consider a web server that uses async/await to handle incoming HTTP requests. With each request running asynchronously, the server doesn’t block while waiting for one task to finish. Instead, it processes multiple requests simultaneously, allowing more efficient handling of high traffic.
Here’s an example of an async HTTP server in Python with asyncio
and the aiohttp
library:
python
from aiohttp import web
import asyncio
async def handle_request(request):
await asyncio.sleep(1) # Simulate a non-blocking I/O task
return web.Response(text="Hello, world!")
app = web.Application()
app.router.add_get('/', handle_request)
web.run_app(app)
This setup allows the server to manage numerous requests concurrently, reducing response times and improving scalability.
Concurrency: Executing Multiple Requests with Node.js and Python’s Asyncio
Concurrency allows programs to perform multiple tasks at once by managing them efficiently through an event loop. In asynchronous programming, the event loop manages multiple tasks by delegating I/O-bound tasks to run in the background, freeing up resources for other tasks. Two common environments for concurrency are Node.js and Python’s asyncio
.
However, concurrency alone doesn’t address the challenges of web scraping when facing issues like rate limiting, CAPTCHAs, or IP blocks. If you’re encountering frequent blocks or scraping limitations in your projects, there’s a tool to help make data extraction efficient and hassle-free.
Having trouble with web scraping challenges and constant blocks on the project you working?
I use Scrapeless to make data extraction easy and efficient, all in one powerful tool.
Try it free today!
Concurrency in Node.js
Node.js, built on the V8 JavaScript engine, uses a single-threaded event loop, which is ideal for asynchronous operations. It employs non-blocking I/O and callback functions for tasks that would otherwise block the thread, making it an efficient choice for applications that require high concurrency.
javascript
const http = require('http');
const server = http.createServer((req, res) => {
setTimeout(() => {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('Hello, world!');
}, 1000); // Non-blocking I/O with setTimeout
});
server.listen(8080, () => {
console.log('Server running at http://127.0.0.1:8080/');
});
Concurrency in Python’s Asyncio
Python’s asyncio
library enables developers to run concurrent tasks by utilizing an event loop that can handle multiple tasks simultaneously. Python’s async/await syntax is particularly helpful here, making it easy to handle tasks like network requests without blocking the program’s flow.
Here’s an example of Python’s asyncio
handling multiple API calls concurrently:
python
import asyncio
import aiohttp
async def fetch_data(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
return await response.text()
async def main():
urls = ['http://example.com/data1', 'http://example.com/data2', 'http://example.com/data3']
tasks = [fetch_data(url) for url in urls]
results = await asyncio.gather(*tasks)
print(results)
asyncio.run(main())
This code fetches data from three URLs concurrently. While one request is waiting for a server response, the event loop continues to process other requests, maximizing the efficiency of the program.
How to Implement Asynchronous Programming Effectively
Implementing asynchronous programming requires planning, as certain tasks are better suited for async execution than others. Here are a few tips to keep in mind:
- Identify I/O-bound tasks: Asynchronous programming is most effective for tasks that involve waiting, such as network requests, file I/O, or database queries.
- Use async libraries: Many libraries support asynchronous operations, such as
aiohttp
for HTTP requests in Python and thefs
module in Node.js for file handling. Using these libraries can improve performance while ensuring compatibility with the async framework. - Error handling: Handling errors in asynchronous programming can be more complex than in synchronous code, especially in cases where tasks may complete out of order. Make use of exception handling within each async task to prevent failures from affecting the entire program.
Conclusion
Asynchronous programming has become indispensable for modern applications that need to handle multiple tasks simultaneously while ensuring high performance and responsiveness. By understanding the differences between synchronous and asynchronous programming, event-driven architecture, and concurrency, developers can build more scalable applications.
Whether you’re building a real-time web server, handling multiple API requests, or optimizing data processing, adopting asynchronous programming techniques can significantly improve your applications’ speed, scalability, and resource usage. As you dive deeper, explore frameworks like Node.js for JavaScript and asyncio
for Python, which provide robust solutions for building efficient asynchronous systems.
At Scrapeless, we only access publicly available data while strictly complying with applicable laws, regulations, and website privacy policies. The content in this blog is for demonstration purposes only and does not involve any illegal or infringing activities. We make no guarantees and disclaim all liability for the use of information from this blog or third-party links. Before engaging in any scraping activities, consult your legal advisor and review the target website's terms of service or obtain the necessary permissions.